Events are “signals” written at specific moments in your song, which the player can detect. As explained here, this is useful to synchronize your production with a music without the coder having to count the frames. A demo can be music-driven!
Events are an 8-bit integer from 0 to 255 (FF
in hex). What it means is up to you. You could simply use 01
and say to the coder “01
, or any other number, means the demo moves forward”. Or something more sophisticated like:
01
means the colors must flash.02
means the screen must wobble.03
means the dots effect must start.04
means the 3D effect must start”.
This is entirely up to you to know what these numbers mean.
Writing events
This is explained in the aforementioned page, but here is a summary. At any times in event tracks, write a number between 01
and FF
.

Nothing happens in AT when writing these. Only the players on the hardware can receive and interpret these.
Receiving events
This is where this get technical. Not all players can naturally handle events. AKG does, but AKM and AKY does not. Don’t worry, there is a trick for it explained below.
Export the song as you would normally do. It contains the events within its data.
In your assembly code (Z80 for example), play the song normally as it is done in the testers provided in the package:
;Main loop. I suppose this runs at 50 Hz.
call PLY_AKG_Play
;Is there any event? 0 means "no effect".
ld a,(PLY_AKG_Event)
or a
jr z,NoEvents
;Ah, there is an event!
cp 1
jr z,FlashColor
cp 2
jr z,WobbleScreen
;... and so on.
NoEvents
;Continue your main code here...
This is only a raw code but it is a starting point (if you handle many events, it would be better to have a pointer table and jump directly on the code instead of chaining many CPs, but optimization is not the purpose of this tutorial!).
This is it if you use the AKG player. If using any other player, you might be embarrassed as none supports events directly. Read on…
Exporting events
If not using AKG player, you will have to handle the events by yourself. Don’t worry it is easy. Let’s pretend that your events were written as this in your song (with a speed of 06
).

First of all, export the events. Go to File > Export > Export events. A dialog opens. For our example, make sure that the source profile is Z80, and source. Then press Export:
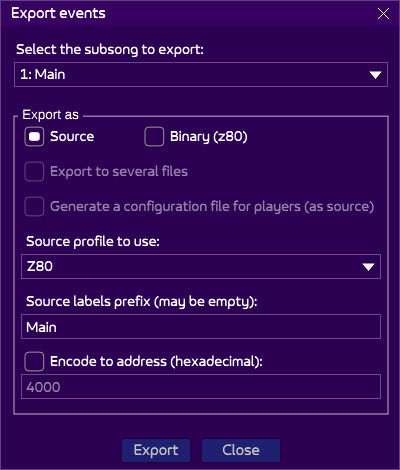
A Z80 source code is generated. Let’s look at it (this example has been cleaned of some comments and labels for cleanliness):
; Events generated by Arkos Tracker 3.
MainEvents
MainEvents_Loop
dw 1 ; Wait for 0 frames.
db 1
dw 42 ; Wait for 41 frames.
db 2
dw 42 ; Wait for 41 frames.
db 1
dw 36 ; Wait for 35 frames.
db 3
dw 54 ; Wait for 53 frames.
db 4
dw 42 ; Wait for 41 frames.
db 5
dw 168 ; Wait for 167 frames.
db 0
dw 0 ; End of sequence.
dw MainEvents_Loop ; Loops here.
This is pretty straightforward. It is only a list of pair of values:
- First, a 16-bit number representing how many frames to wait -1:
- 50 means wait for 49 frames before reading the event number.
- 49 means wait for 48 frames before reading the event number.
- …
- 2 means wait for 1 frame before reading the event number.
- 1 means “don’t wait”: read the event.
- 0 means “end of list”, followed by the address where to loop to. Continue the parsing.
- Then, a 8-bit integer of the event number. If 0, it means no event, simply continues the parsing.
After reading a valid event (>0), you can stop the parsing for this frame.
In the unlikely event that more than 65535 frames must be waited, and thus a 16-bit number is not enough, then more than one wait is encoded, with an event number of 0 (meaning no event, continue parsing). There is actually no special code to perform if you followed the rules described above.
There is no code provided to parse this, I will leave it to you (unless I am asked to do it!), as it is very simple.